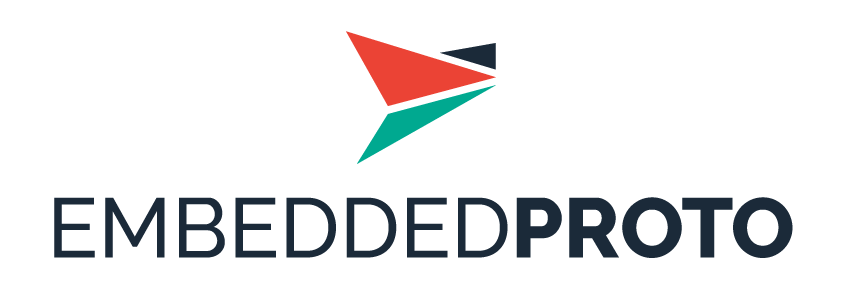 |
EmbeddedProto
2.0.0
EmbeddedProto is a C++ Protocol Buffer implementation specifically suitable for microcontrollers.
|
Go to the documentation of this file.
31 #ifndef _WIRE_FORMATTER_H_
32 #define _WIRE_FORMATTER_H_
40 #include <type_traits>
52 static constexpr uint8_t VARINT_SHIFT_N_BITS = 7;
55 static constexpr uint8_t VARINT_MSB_BYTE = 0x80;
64 static constexpr int32_t constexpr_ceil(
float num)
66 return (
static_cast<float>(
static_cast<int32_t
>(num)) == num)
67 ?
static_cast<int32_t
>(num)
68 :
static_cast<int32_t
>(num) + ((num > 0) ? 1 : 0);
93 template<
class INT_TYPE>
96 static_assert(std::is_same<INT_TYPE, int32_t>::value ||
97 std::is_same<INT_TYPE, int64_t>::value,
"Wrong type passed to ZigZagEncode.");
99 typedef typename std::make_unsigned<INT_TYPE>::type UINT_TYPE;
100 constexpr uint8_t N_BITS_TO_ZIGZAG = std::numeric_limits<UINT_TYPE>::digits - 1;
102 return (
static_cast<UINT_TYPE
>(n) << 1) ^
static_cast<UINT_TYPE
>(n >> N_BITS_TO_ZIGZAG);
112 template<
class UINT_TYPE>
115 static_assert(std::is_same<UINT_TYPE, uint32_t>::value ||
116 std::is_same<UINT_TYPE, uint64_t>::value,
"Wrong type passed to ZigZagDecode.");
118 typedef typename std::make_signed<UINT_TYPE>::type INT_TYPE;
120 return static_cast<INT_TYPE
>((n >> 1) ^ (~(n & 1) + 1));
131 return ((field_number << 3) |
static_cast<uint32_t
>(type));
139 template<
class UINT_TYPE>
143 static_assert(std::is_same<UINT_TYPE, uint32_t>::value ||
144 std::is_same<UINT_TYPE, uint64_t>::value,
"Wrong type passed to SerialzieFixedNoTag.");
152 for(uint8_t i = 0; (i < std::numeric_limits<UINT_TYPE>::digits) && result; i += 8) {
154 result = buffer.
push(
static_cast<uint8_t
>((value >> i) & 0x00FF));
160 template<
class INT_TYPE>
163 static_assert(std::is_same<INT_TYPE, int32_t>::value ||
164 std::is_same<INT_TYPE, int64_t>::value,
"Wrong type passed to SerialzieSFixedNoTag.");
166 typedef typename std::make_unsigned<INT_TYPE>::type UINT_TYPE;
175 void* pVoid =
static_cast<void*
>(&value);
176 uint32_t* fixed =
static_cast<uint32_t*
>(pVoid);
184 void* pVoid =
static_cast<void*
>(&value);
185 uint64_t* fixed =
static_cast<uint64_t*
>(pVoid);
195 template<
class INT_TYPE>
198 typedef typename std::make_unsigned<INT_TYPE>::type UINT_TYPE;
207 template<
class UINT_TYPE>
218 template<
class INT_TYPE>
294 const uint8_t
byte = value ? 0x01 : 0x00;
336 type =
static_cast<WireType>(temp_value & 0x07);
337 id = (temp_value >> 3);
347 template<
class UINT_TYPE>
350 static_assert(std::is_same<UINT_TYPE, uint32_t>::value ||
351 std::is_same<UINT_TYPE, uint64_t>::value,
"Wrong type passed to DeserializeUInt.");
356 template<
class INT_TYPE>
359 static_assert(std::is_same<INT_TYPE, int32_t>::value ||
360 std::is_same<INT_TYPE, int64_t>::value,
"Wrong type passed to DeserializeInt.");
366 value =
static_cast<INT_TYPE
>(uint_value);
371 template<
class INT_TYPE>
374 static_assert(std::is_same<INT_TYPE, int32_t>::value ||
375 std::is_same<INT_TYPE, int64_t>::value,
"Wrong type passed to DeserializeSInt.");
389 static_assert(std::is_same<TYPE, uint32_t>::value ||
390 std::is_same<TYPE, uint64_t>::value,
"Wrong type passed to DeserializeFixed.");
398 for(uint8_t i = 0; (i < std::numeric_limits<TYPE>::digits) && result;
399 i += std::numeric_limits<uint8_t>::digits)
401 result = buffer.
pop(
byte);
404 temp_value |= (
static_cast<TYPE
>(byte) << i);
421 template<
class STYPE>
424 static_assert(std::is_same<STYPE, int32_t>::value ||
425 std::is_same<STYPE, int64_t>::value,
"Wrong type passed to DeserializeSFixed.");
427 typedef typename std::make_unsigned<STYPE>::type USTYPE;
428 USTYPE temp_unsigned_value = 0;
432 value =
static_cast<STYPE
>(temp_unsigned_value);
440 uint32_t temp_value = 0;
445 const void* pVoid =
static_cast<const void*
>(&temp_value);
446 const float* pFloat =
static_cast<const float*
>(pVoid);
454 uint64_t temp_value = 0;
459 const void* pVoid =
static_cast<const void*
>(&temp_value);
460 const double* pDouble =
static_cast<const double*
>(pVoid);
472 value =
static_cast<bool>(byte);
481 template<
class ENUM_TYPE>
484 static_assert(std::is_enum<ENUM_TYPE>::value,
"No enum given to DeserializeEnum parameter value.");
489 value =
static_cast<ENUM_TYPE
>(temp_value);
504 template<
class UINT_TYPE>
507 static_assert(std::is_same<UINT_TYPE, uint32_t>::value ||
508 std::is_same<UINT_TYPE, uint64_t>::value,
509 "Wrong type passed to SerializeVarint.");
511 bool memory_free =
true;
512 while((value >= VARINT_MSB_BYTE) && memory_free)
514 memory_free = buffer.
push(
static_cast<uint8_t
>(value | VARINT_MSB_BYTE));
515 value >>= VARINT_SHIFT_N_BITS;
517 memory_free = buffer.
push(
static_cast<uint8_t
>(value));
529 template<
class UINT_TYPE>
532 static_assert(std::is_same<UINT_TYPE, uint32_t>::value ||
533 std::is_same<UINT_TYPE, uint64_t>::value,
534 "Wrong type passed to DeserializeVarint.");
538 static constexpr uint8_t N_BYTES_IN_VARINT =
static_cast<uint8_t
>(constexpr_ceil(
539 std::numeric_limits<UINT_TYPE>::digits
540 /
static_cast<float>(VARINT_SHIFT_N_BITS)));
542 UINT_TYPE temp_value = 0;
544 bool result = buffer.
pop(
byte);
546 for(uint8_t i = 0; (i < N_BYTES_IN_VARINT) && result; ++i)
548 temp_value |=
static_cast<UINT_TYPE
>(
byte & (~VARINT_MSB_BYTE)) << (i * VARINT_SHIFT_N_BITS);
549 if(
byte & VARINT_MSB_BYTE)
552 result = buffer.
pop(
byte);
The pure virtual definition of a message buffer to read from.
Definition: ReadBufferInterface.h:43
@ INVALID_WIRETYPE
When reading a Wiretype from the tag we got an invalid value.
@ NO_ERRORS
No errors have occurred.
virtual bool push(const uint8_t byte)=0
Push a single byte into the buffer.
The pure virtual definition of a message buffer used for writing .
Definition: WriteBufferInterface.h:46
Error
This enumeration defines errors which can occur during serialization and deserialization.
Definition: Errors.h:38
@ END_OF_BUFFER
While trying to read from the buffer we ran out of bytes tor read.
virtual bool pop(uint8_t &byte)=0
Obtain the value of the oldest byte in the buffer and remove it from the buffer.
@ BUFFER_FULL
The write buffer is full, unable to push more bytes in to it.