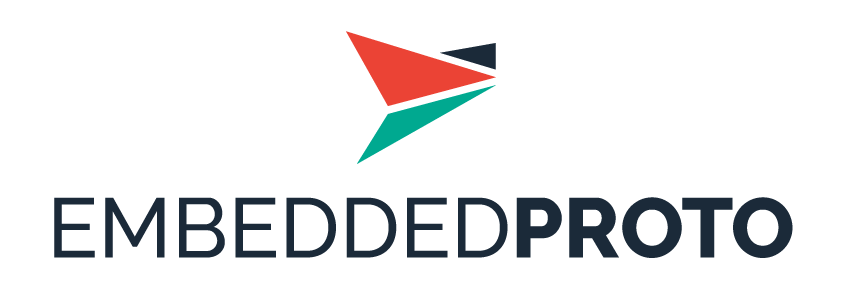 |
EmbeddedProto
2.0.0
EmbeddedProto is a C++ Protocol Buffer implementation specifically suitable for microcontrollers.
|
Go to the documentation of this file.
31 #ifndef _REPEATED_FIELD_SIZE_H_
32 #define _REPEATED_FIELD_SIZE_H_
50 template<
class DATA_TYPE, u
int32_t MAX_LENGTH>
53 static constexpr uint32_t BYTES_PER_ELEMENT =
sizeof(DATA_TYPE);
80 uint32_t
get_length()
const override {
return current_length_; }
86 uint32_t
get_size()
const override {
return BYTES_PER_ELEMENT * current_length_; }
89 uint32_t
get_max_size()
const override {
return BYTES_PER_ELEMENT * MAX_LENGTH; }
91 DATA_TYPE&
get(uint32_t index)
override
93 uint32_t limited_index = std::min(index, MAX_LENGTH-1);
95 if(limited_index >= current_length_) {
96 current_length_ = limited_index + 1;
98 return data_[limited_index];
101 const DATA_TYPE&
get_const(uint32_t index)
const override
103 uint32_t limited_index = std::min(index, MAX_LENGTH-1);
104 return data_[limited_index];
107 void set(uint32_t index,
const DATA_TYPE& value)
override
109 uint32_t limited_index = std::min(index, MAX_LENGTH-1);
111 if(limited_index >= current_length_) {
112 current_length_ = limited_index + 1;
114 data_[limited_index] = value;
120 if(MAX_LENGTH >= length)
122 const DATA_TYPE* d = data;
123 for(uint32_t i = 0; i < length; ++i)
128 current_length_ = length;
140 if(MAX_LENGTH > current_length_)
142 data_[current_length_] = value;
154 for(uint32_t i = 0; i < current_length_; ++i)
164 uint32_t current_length_;
167 DATA_TYPE data_[MAX_LENGTH];
172 #endif // End of _REPEATED_FIELD_SIZE_H_
Error add(const DATA_TYPE &value) override
Append a value to the end of the array.
Definition: RepeatedFieldFixedSize.h:137
Class template that specifies the interface of an arry with the data type.
Definition: RepeatedField.h:50
DATA_TYPE & get(uint32_t index) override
Get a reference to the value at the given index.
Definition: RepeatedFieldFixedSize.h:91
@ NO_ERRORS
No errors have occurred.
~RepeatedFieldFixedSize() override=default
uint32_t get_size() const override
Obtain the total number of bytes currently stored in the array.
Definition: RepeatedFieldFixedSize.h:86
Error
This enumeration defines errors which can occur during serialization and deserialization.
Definition: Errors.h:38
const DATA_TYPE & get_const(uint32_t index) const override
Get a constant reference to the value at the given index.
Definition: RepeatedFieldFixedSize.h:101
uint32_t get_max_length() const override
Obtain the maximum number of DATA_TYPE items which can at most be stored in the array.
Definition: RepeatedFieldFixedSize.h:83
Error set_data(const DATA_TYPE *data, const uint32_t length) override
Given a different array of known length copy that data into this object.
Definition: RepeatedFieldFixedSize.h:117
void set(uint32_t index, const DATA_TYPE &value) override
Set the value at the given index.
Definition: RepeatedFieldFixedSize.h:107
void clear() override
Remove all data in the array and set it to the default value.
Definition: RepeatedFieldFixedSize.h:152
@ ARRAY_FULL
The array is full, it is not possible to push more items in it.
RepeatedFieldFixedSize()
Definition: RepeatedFieldFixedSize.h:57
A template class that actually holds some data.
Definition: RepeatedFieldFixedSize.h:51
uint32_t get_max_size() const override
Obtain the maximum number of bytes which can at most be stored in the array.
Definition: RepeatedFieldFixedSize.h:89
RepeatedFieldFixedSize< DATA_TYPE, MAX_LENGTH > & operator=(const RepeatedFieldFixedSize< DATA_TYPE, MAX_LENGTH > &rhs)
Assign one repieted field to the other, but only when the length and type matches.
Definition: RepeatedFieldFixedSize.h:67
uint32_t get_length() const override
Obtain the total number of DATA_TYPE items in the array.
Definition: RepeatedFieldFixedSize.h:80