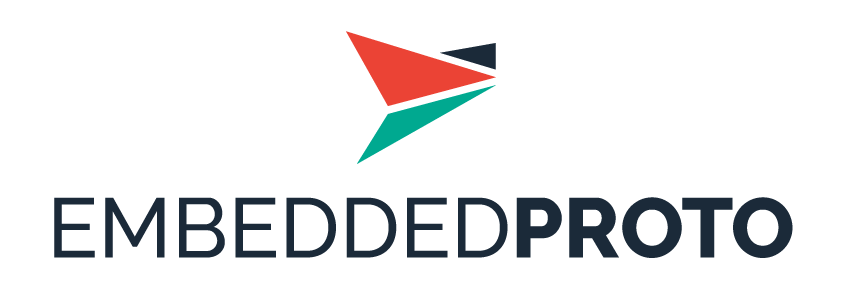 |
EmbeddedProto
2.0.0
EmbeddedProto is a C++ Protocol Buffer implementation specifically suitable for microcontrollers.
|
Go to the documentation of this file.
31 #ifndef _FIELD_STRING_BYTES_H_
32 #define _FIELD_STRING_BYTES_H_
39 #include <type_traits>
50 template<u
int32_t MAX_LENGTH,
class DATA_TYPE>
53 static_assert(std::is_same<uint8_t, DATA_TYPE>::value || std::is_same<char, DATA_TYPE>::value,
54 "This class only supports unit8_t or chars.");
87 DATA_TYPE&
get(uint32_t index)
89 uint32_t limited_index = std::min(index, MAX_LENGTH-1);
94 return data_[limited_index];
105 uint32_t limited_index = std::min(index, MAX_LENGTH-1);
106 return data_[limited_index];
128 Error set(
const DATA_TYPE* data,
const uint32_t length)
131 if(MAX_LENGTH >= length)
134 memcpy(
data_, data, length);
176 const void* void_pointer =
static_cast<const void*
>(&(
data_[0]));
177 const uint8_t* byte_pointer =
static_cast<const uint8_t*
>(void_pointer);
191 if(MAX_LENGTH >= availiable)
236 template<u
int32_t MAX_LENGTH>
245 const uint32_t rhs_MAX_LENGTH = strlen(rhs);
257 template<u
int32_t MAX_LENGTH>
268 #endif // End of _FIELD_STRING_BYTES_H_
Error serialize(WriteBufferInterface &buffer) const override
Definition: FieldStringBytes.h:173
uint32_t current_length_
Number of item in the data array.
Definition: FieldStringBytes.h:227
Error set(const DATA_TYPE *data, const uint32_t length)
Definition: FieldStringBytes.h:128
The pure virtual definition of a message buffer to read from.
Definition: ReadBufferInterface.h:43
const DATA_TYPE * get_const() const
Get a constant pointer to the first element in the array.
Definition: FieldStringBytes.h:77
@ NO_ERRORS
No errors have occurred.
FieldStringBytes()
Definition: FieldStringBytes.h:58
virtual bool push(const uint8_t byte)=0
Push a single byte into the buffer.
The pure virtual definition of a message buffer used for writing .
Definition: WriteBufferInterface.h:46
virtual uint32_t get_available_size() const =0
Obtain the total number of bytes still available in the buffer.
Error
This enumeration defines errors which can occur during serialization and deserialization.
Definition: Errors.h:38
void clear() override
Reset the field to it's initial value.
Definition: FieldStringBytes.h:218
uint32_t get_length() const
Obtain the number of characters in the string right now.
Definition: FieldStringBytes.h:71
DATA_TYPE & get(uint32_t index)
Get a reference to the value at the given index.
Definition: FieldStringBytes.h:87
@ END_OF_BUFFER
While trying to read from the buffer we ran out of bytes tor read.
DATA_TYPE data_[MAX_LENGTH]
The text.
Definition: FieldStringBytes.h:230
virtual bool pop(uint8_t &byte)=0
Obtain the value of the oldest byte in the buffer and remove it from the buffer.
Definition: FieldStringBytes.h:51
void operator=(const char *const &&rhs)
Definition: FieldStringBytes.h:243
Definition: FieldStringBytes.h:237
uint32_t get_max_length() const
Obtain the maximum number characters in the string.
Definition: FieldStringBytes.h:74
virtual ~FieldBytes()=default
@ ARRAY_FULL
The array is full, it is not possible to push more items in it.
const DATA_TYPE & get_const(uint32_t index) const
Get a constant reference to the value at the given index.
Definition: FieldStringBytes.h:103
Definition: FieldStringBytes.h:258
virtual ~FieldString()=default
const DATA_TYPE & operator[](uint32_t index) const
Get a constant reference to the value at the given index.
Definition: FieldStringBytes.h:125
Error serialize_with_id(uint32_t field_number, WriteBufferInterface &buffer) const override
Definition: FieldStringBytes.h:144
Error deserialize(ReadBufferInterface &buffer) override
Definition: FieldStringBytes.h:185
@ BUFFER_FULL
The write buffer is full, unable to push more bytes in to it.
virtual ~FieldStringBytes()
Definition: FieldStringBytes.h:65
Definition: FieldStringBytes.h:48
DATA_TYPE & operator[](uint32_t index)
Get a reference to the value at the given index.
Definition: FieldStringBytes.h:117